Java Beginners Tutotrial
RequestDispatcher methods with examples in Servlet
How to use load-on-startup tag in web.xml file with Example
How to create and run Servlet in Eclipse IDE
How to run JSP in Eclipse IDE using Apache Tomcat Server
How to configure Apache Tomcat Server in Eclipse IDE
How to fix Dynamic Web Project missing in Eclipse issue
Solution – No Apache Tomcat Adapter option in Eclipse IDE
Solution – The superclass “javax.servlet.http.HttpServlet” was not found on the Java Build Path
Java tutorial for beginners with examples
This tutorial would help you learn java like a pro. We have shared 500+ tutorials on various topics of java including basic java concepts, java programming examples and flow diagrams. This is a consolidated tutorial that provides you the links of all the key concepts in a systematic order starting from beginner’s level to the advance topics for java experts. Whether you are a college student looking for learn java basics or a company employee looking for java advance topics for building an application in java, this tutorial would definitely be useful for you. Let’s start learning…
Java introduction
Start from here. An introduction to java along with java fundamentals with examples.
1) Introduction to Java 2) First Java Program 3) Java Virtual Machine(JVM) Basics | 4) For loop 5) While loop 6) do-while loop |
Try out few Java Programs – Only for beginners
Got a basic idea about java? Try out these programs. If you are learning java, you would probably get few of them in you assignments.
Java Program to:
Java Program to:
Object oriented programming concepts
Java is an object oriented programming language and this is one of the main feature of java. I have explained each and every concept of oops in detail along with examples. Here are the most read tutorials in OOPs concepts category. To get the full list of OOPS concepts tutorial, refer this article: OOPs concepts in java.
1) Constructor 2) Polymorphism 3) Method overloading 4) Method overriding 5) Types of polymorphism 6) Inheritance 7) Types of inheritance 8) Encapsulation 9) Static and dynamic binding | 10) Static Keyword 11) Inner classes 12) Abstract class and methods 13) Interface 14) Access modifiers 15) Packages 16) final keyword 17) super keyword |
Java Tuorials
List of Miscellaneous tutorials.
1) Java Exception handling 2) Java String – Collection of 45+ tutorials on string handling methods in java with examples. 3) Multithreading in Java 4) Java I/O tutorials – Collection of Input/Output related tutorials in java. | 5) Java Annotations 6) Java autoboxing and unboxing 7) Java Regular expressions Tutorial 8) Java Enum |
Java – Collections
Java Collections Framework Tutorials – 100+ tutorials on collections classes and interfaces. Few popular tutorials from this category are:
1) Java ArrayList 2) Java LinkedList 3) Java Vector 4) Java HashSet 5) Java TreeSet | 6) Java LinkedHashSet 7) Java HashMap 8) Java TreeMap 9) Java LinkedHashMap 10) Java Hashtable |
OOPs Basics
Java Collections Framework Tutorials
The Java Collections Framework is a collection of interfaces and classes which helps in storing and processing the data efficiently. This framework has several useful classes which have tons of useful functions which makes a programmer task super easy. I have written several tutorials on Collections and below are the links of those. All the tutorials are shared with examples and source codes to help you understand better.
Collections Framework hierarchy
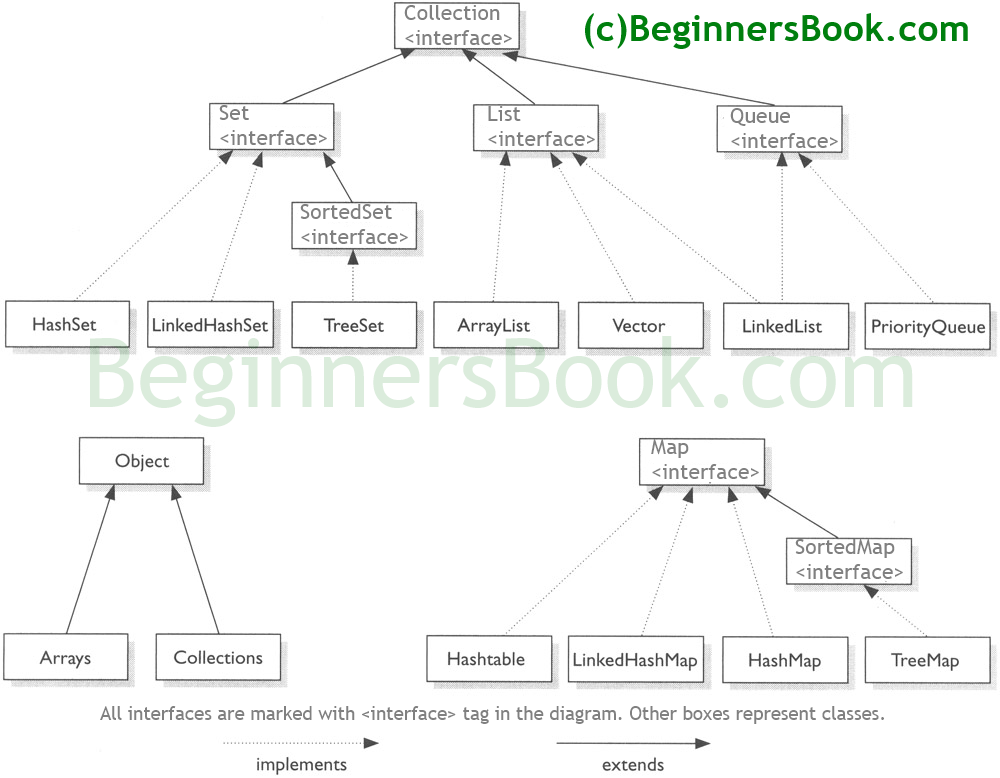
List
A List is an ordered Collection (sometimes called a sequence). Lists may contain duplicate elements. Elements can be inserted or accessed by their position in the list, using a zero-based index.
Set
A Set is a Collection that cannot contain duplicate elements. There are three main implementations of Set interface: HashSet, TreeSet, and LinkedHashSet. HashSet, which stores its elements in a hash table, is the best-performing implementation; however it makes no guarantees concerning the order of iteration. TreeSet, which stores its elements in a red-black tree, orders its elements based on their values; it is substantially slower than HashSet. LinkedHashSet, which is implemented as a hash table with a linked list running through it, orders its elements based on the order in which they were inserted into the set (insertion-order).
Map
A Map is an object that maps keys to values. A map cannot contain duplicate keys. There are three main implementations of Map interfaces: HashMap, TreeMap, and LinkedHashMap.
HashMap: it makes no guarantees concerning the order of iteration
TreeMap: It stores its elements in a red-black tree, orders its elements based on their values; it is substantially slower than HashMap.
LinkedHashMap: It orders its elements based on the order in which they were inserted into the set (insertion-order).
HashMap: it makes no guarantees concerning the order of iteration
TreeMap: It stores its elements in a red-black tree, orders its elements based on their values; it is substantially slower than HashMap.
LinkedHashMap: It orders its elements based on the order in which they were inserted into the set (insertion-order).
Iterator/ListIterator
Both Iterator and ListIterator are used to iterate through elements of a collection class. Using Iterator we can traverse in one direction (forward) while using ListIterator we can traverse the collection class on both the directions(backward and forward). To know more differences between these two refer this article: Difference between Iterator and ListIterator.
Java I/O tutorial with examples
I have written several tutorials on Java I/O. You can find out the links of all the tutorials below. The tutorials are explained with the help of very basic and simple examples so that even a beginner can learn with ease. I will continue to write more tutorials on I/O and will add the links below.
Create File
Read File
1) How to read a file in java using BufferedInputStream
2) How to read a file in java using BufferedReader
2) How to read a file in java using BufferedReader
Write/Append File
1) How to write to a file in java using FileOutputStream
2) How to write to file in Java using BufferedWriter
3) Append to a file in java using BufferedWriter, PrintWriter, FileWriter
2) How to write to file in Java using BufferedWriter
3) Append to a file in java using BufferedWriter, PrintWriter, FileWriter
Delete/Rename File
1) How to delete file in Java using delete() Method
2) How to rename file in Java using renameTo() method
2) How to rename file in Java using renameTo() method
File compression
Misc
JSON Tutorial: Learn JSON in 10 Minutes
JSON stands for JavaScript Object Notation. JSON objects are used for transferring data between server and client, XML serves the same purpose. However JSON objects have several advantages over XML and we are going to discuss them in this tutorial along with JSON concepts and its usages.
Let’s have a look at the piece of a JSON data: It basically has key-value pairs.
var chaitanya = { "firstName" : "Chaitanya", "lastName" : "Singh", "age" : "28" };
Features of JSON:
- It is light-weight
- It is language independent
- Easy to read and write
- Text based, human readable data exchange format
Why use JSON?
Standard Structure: As we have seen so far that JSON objects are having a standard structure that makes developers job easy to read and write code, because they know what to expect from JSON.
Light weight: When working with AJAX, it is important to load the data quickly and asynchronously without requesting the page re-load. Since JSON is light weighted, it becomes easier to get and load the requested data quickly.
Scalable: JSON is language independent, which means it can work well with most of the modern programming language. Let’s say if we need to change the server side language, in that case it would be easier for us to go ahead with that change as JSON structure is same for all the languages.
JSON vs. XML
Let see how JSON and XML look when we store the records of 4 students in a text based format so that we can retrieve it later when required.
JSON style:
{"students":[ {"name":"John", "age":"23", "city":"Agra"}, {"name":"Steve", "age":"28", "city":"Delhi"}, {"name":"Peter", "age":"32", "city":"Chennai"}, {"name":"Chaitanya", "age":"28", "city":"Bangalore"} ]}
XML style:
<students> <student> <name>John</name> <age>23</age> <city>Agra</city> </student> <student> <name>Steve</name> <age>28</age> <city>Delhi</city> </student> <student> <name>Peter</name> <age>32</age> <city>Chennai</city> </student> <student> <name>Chaitanya</name> <age>28</age> <city>Bangalore</city> </student> </students>
As you can clearly see JSON is much more light-weight compared to XML. Also, in JSON we take advantage of arrays that is not available in XML.
JSON data structure types and how to read them:
- JSON objects
- JSON objects in array
- Nesting of JSON objects
1) JSON objects:
var chaitanya = { "name" : "Chaitanya Singh", "age" : "28", "website" : "beginnersbook" };
The above text creates an object that we can access using the variable chaitanya. Inside an object we can have any number of key-value pairs like we have above. We can access the information out of a JSON object like this:
document.writeln("The name is: " +chaitanya.name); document.writeln("his age is: " + chaitanya.age); document.writeln("his website is: "+ chaitanya.website);
2) JSON objects in array
In the above example we have stored the information of one person in a JSON object suppose we want to store the information of more than one person; in that case we can have an array of objects.
var students = [{ "name" : "Steve", "age" : "29", "gender" : "male" }, { "name" : "Peter", "age" : "32", "gender" : "male" }, { "name" : "Sophie", "age" : "27", "gender" : "female" }];
To access the information out of this array, we do write the code like this:
document.writeln(students[0].age); //output would be: 29 document.writeln(students[2].name); //output: Sophie
You got the point, right? Let’s carry on with the next type.
3) Nesting of JSON objects:
Another way of doing the same thing that we have done above.
var students = { "steve" : { "name" : "Steve", "age" : "29", "gender" : "male" }, "pete" : { "name" : "Peter", "age" : "32", "gender" : "male" }, "sop" : { "name" : "Sophie", "age" : "27", "gender" : "female" } }
Do like this to access the info from above nested JSON objects:
document.writln(students.steve.age); //output: 29 document.writeln(students.sop.gender); //output: female
JSON & JavaScript:
JSON is considered as a subset of JavaScript but that does not mean that JSON cannot be used with other languages. In fact it works well with PHP, Perl, Python, Ruby, Java, Ajax and many more.
Just to demonstrate how JSON can be used along with JavaScript, here is an example:
If you have gone though the above tutorial, you are familiar with the JSON structures. A JSON file type is .json
If you have gone though the above tutorial, you are familiar with the JSON structures. A JSON file type is .json
How to read data from json file and convert it into a JavaScript object?
We have two ways to do this.
1) Using eval function, but this is not suggested due to security reasons (malicious data can be sent from the server to the client and then eval in the client script with harmful effects).
2) Using JSON parser: No security issues plus it is faster than eval. Here is how to use it:
We have two ways to do this.
1) Using eval function, but this is not suggested due to security reasons (malicious data can be sent from the server to the client and then eval in the client script with harmful effects).
2) Using JSON parser: No security issues plus it is faster than eval. Here is how to use it:
var chaitanya = { "name" : "Chaitanya Singh", "age" : "28", "website" : "beginnersbook" };
We are converting the above JSON object to javascript object using JSON parser:
var myJSObject = JSON.parse(chaitanya);
How to convert JavaScript object to JSON text?
By using method stringify
By using method stringify
var jsonText= JSON.stringify(myJSObject);
I guess the 10 minutes are over. Bookmark this page as I will be adding more tutorials on JSON and will add the links to those tutorials here.
JSP tutorial for beginners with examples – Java Server Pages
Java Server Pages (JSP) is a server side technology for developing dynamic web pages. This is mainly used for implementing presentation layer (GUI Part) of an application. A complete JSP code is more like a HTML with bits of java code in it. JSP is an extension of servlets and every JSP page first gets converted into servlet by JSP container before processing the client’s request.
Below are the links of tutorial shared on JSP. If you are beginner then read them in the given sequence to understand the technology in a better way.
Overview
Elements of JSP
Directives
JSP directives are used for controlling the processing of a JSP page. Directives provide information to the server on how the page should be processed.
- Page and Taglib directive – Page directive and it’s attributes in detail along with the introduction of taglib directive.
- Include Directive – Includes the page to the current page during JSP to servlet translation.
- Include directive with parameters – This guide will help you learn how to pass parameters and their values to another page while using include directive.
Scriptlets
We can use java code in JSP using scriptlets. The JSP container moves the scipetlet content into the _jspService() method which is available to the server during processing of the request.
Scriptlet tutorial – It will help you learn how to use scriptlet in JSP.
Action Tags
They are used for performing an action during request processing phase of JSP life cycle.
- Action tags introduction – An introduction to all the available action tags in JSP
- Include action tag – This tag is used for including a file to the current JSP during request processing (dynamically).
- Include action tag with parameters – <jsp:include> and <jsp:param> example for passing parameters while including another page to the current page.
- forward action tag – It is used for redirecting the request to another page.
- useBean, setProperty and getProperty action tags – Learn how to use bean class in JSP.
Expressions
Expression tag – Mainly used for displaying the content on the browser by sending back the result to client through response object.
Declarations
Declaration tag – Learn how to declare variables and methods in JSP.
JSP Implicit Objects
These objects are created by JSP container while translating the JSP page to Servlet. These objects are present inside service methods so we can directly use them without declaration.
- Introduction to implicit objects
- out implicit object
- request implicit object
- response implicit object
- session implicit object
- application implicit object
- exception implicit object
- pageContext implicit object
- config implicit object
Expression language(EL) in JSP
Expression language(EL) – We can easily access the data of variables, bean components and expressions using Expression language. Must read tutorial for JSP beginners.
Exception handling
Exception handling in JSP – A complete tutorial to learn exception handling in JSP. We have shared two methods to handle exceptions.
Custom Tags
To find out more tutorials on JSP refer the archive here.
More tutorials »
JSTL functions and Core Tags- JSTL(JSP Standard Tag Lib) Tutorial
JSTL stands for JSP standard tag Library which is a collection of very useful core tags and functions. These tags and functions will help you write JSP code efficiently.
JSTL Core Tags
Below is the collection of tutorials on JSTL core tags. Each tutorial is explained with the help of screenshots and proper examples. The following line of statement must be present in your JSP in order to use the JSTL core Tags.
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
- <c:out> tag: It is used for displaying the content on client after escaping XML and HTML markup tags. Main attributes are default and escapeXML.
- <c:set> tag: This tag is useful for setting up a variable value in a specified scope. It basically evaluates an expression and sets the result in given variable.
- <c:remove> tag: It is used for removing an attribute from a specified scope or from all scopes (page, request, session and application). By default removes from all.
- <c: if> tag: This JSTL core tag is used for testing conditions. There are two other optional attributes for this tag which are var and scope, test is mandatory.
- <c:choose> tag: It’s like switch statement in Java.
- <c:when> tag: It’s like case statement in Java.
- <c:otherwise> tag: It works like default attribute in switch-case statements.
- <c:catch>tag: This tag is used in exception handling. In this post we have discussed exception handling using <c:catch> core tag.
- <c:import> tag: This JSTL core tag is used for importing the content from another file/page to the current JSP page. Attributes – var, URL and scope.
- <c:forEach> tag: This tag in JSTL is used for executing the same set of statements for a finite number of times.
- <c:forTokens> tag: It is used for iteration but it only works with delimiter.
- <c:param> tag: This JSTL tag is mostly used with <c:url> and <c:redirect> tags. It adds parameter and their values to the output of these tags.
- <c:url> tag: It is used for url formatting or url encoding. It converts a relative url into a application context’s url. Optional attributes var, context and scope.
- <c:redirect> tag: It is used for redirecting the current page to another URL, provide the relative address in the URL attribute of this tag and the page will be redirected to the url.
JSTL Functions
Following are the tutorial links for useful JSTL functions with examples. Following Taglib directive should be included in the JSP page in order to use the JSTL functions.
<%@ taglib prefix="fn" uri="http://java.sun.com/jsp/jstl/functions" %>
- fn:contains function: This function checks whether the given string is present in the input as sub-string. It does a case sensitive check.
- fn:containsIgnoreCase(): It does a case insensitive check to see whether the provided string is a sub-string of input.
- fn:indexOf(): It is used for finding out the start position of a string in the provided string. Function returns -1 when string is not found in the input.
- fn:escapeXML(): It is used for HTML/XML character escaping which means it treats html/xml tags as a string. Similar to the escapeXml attribute of <c:out> tag.
- fn:join() and fn:split() functions: JSTL functions: fn:join() concatenates the strings with a given separator and returns the output string. fn:split() splits a given string into an array of substrings.
- fn:length(): The JSTL function fn:length() is used for computing the length of a string or to find out the number of elements in a collection. It returns the length of the object.
- fn:startsWith(): It checks the specified string is a prefix of given string.
- fn:endsWith(): fn:endsWith() JSTL function is used for checking the suffix of a string. It checks whether the given string ends with a particular string.
- fn:substring(): This JSTL function is used for getting a substring from the provided string.
- fn:substringAfter(): It is used for getting a substring which is present in the input string before a specified string.
- fn:substringBefore(): It gets a substring from input which comes after a specified string.
- fn:trim(): JSTL Function fn:trim() removes spaces from beginning and end of a string and function.
- fn:toUpperCase(): It is just opposite of fn:toLowerCase() function. It converts input string to a uppercase string.
- fn:toLowerCase(): This function is used for converting an input string to a lower case string.
- fn:replace(): fn:replace() function search for a string in the input and replace it with the provided string. It does case sensitive processing.
To find out more tutorials on JSTL refer the archive here.
Best java tutorial.
ReplyDeleteThanks,
https://www.flowerbrackets.com/java-program-to-find-circumference-of-a-circle/
A complete java tutorial for beginners.
ReplyDeleteThanks,
https://www.flowerbrackets.com/arraylist-in-java/
best java tutorial for beginners.
ReplyDeleteThanks,
https://www.flowerbrackets.com/hashset-in-java/
Nice article . Thanks very much for sharing such good post. one more good java
ReplyDeleteresource https://www.javavogue.com/2015/02/the-interface-and-class-hierarchy-for-collection/
One of the best java beginners tutorial.
ReplyDeletehttps://www.flowerbrackets.com/static-keyword-in-java/